This tutorial we going to implement PHP infinite scrolling without page reload using PHP, AJAX, MySQL and jQuery. That helpful for big post website and also useful for pagination as well, which gives great user experience. So let’s begin with create MySQL table and dumb some data.
Create MySQL Table
CREATE TABLE `infinite_scoll` (
`id` int(11) NOT NULL,
`post_title` varchar(255) NOT NULL,
`post_desc` text NOT NULL,
`date_time` datetime NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
ALTER TABLE `infinite_scoll`
ADD PRIMARY KEY (`id`);
ALTER TABLE `infinite_scoll`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT
Let’s have create DB connection file.
Create config.php file
<?php
$servername = "localhost";
$username = "root";
$password = "";
$db = "test";
$conn = mysqli_connect($servername, $username, $password, $db);
// Check The Connection
if (mysqli_connect_errno() ){
echo "Database Connection Failed: " . mysqli_connect_error();
die;
}
mysqli_set_charset($conn,"utf8");
?>
Create index.php file for displaying post we use mysql query to get post from table and call JS file to get data and append it on page.
<?php
include_once('config.php');
$sql="SELECT * FROM infinite_scoll WHERE 1 ORDER BY id DESC LIMIT 10";
$row = mysqli_query($conn,$sql);
?>
<!DOCTYPE html>
<html>
<head>
<title>PHP Infinite scroll</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" />
</head>
<body>
<div class="container">
<div class="row" id="post-data">
<?php include_once("homedata.php"); ?>
</div>
</div>
<div class="ajax-load text-center" style="display:none">
<p><img src="img/loader.gif" style="width: 35px;">Loading More post</p>
</div>
<div class="ajax-load-done text-center" style="display:none">
<p>No more post.</p>
</div>
<script>
loaded = true;
var scrolled = false;
$(window).scroll(function() {
if ($(window).scrollTop() >= $(document).height() - $(window).height() - 70) {
if (!scrolled) {
scrolled = true;
var last_id = $(".post-id:last").attr("id");
loadMoreData(last_id);
}
}
});
function showLoader(){
$('.ajax-load').show();
}
function hideLoader(){
$('.ajax-load').hide();
}
function loadMoreData(last_id){
loaded = false; //<----- change it to false as soon as start ajax call
var idArray = [];
$.ajax({
url: './ajax-checker.php',
type: "post",
data:{last_id_sp:last_id},
beforeSend: function()
{
showLoader();
}
})
.done(function(data){
scrolled = false;
if(data==1){
hideLoader();
$('.ajax-load-done').show();
loaded = true; //<--- again change it to true
}else{
hideLoader();
$("#post-data").append(data);
loaded = true; //<--- again change it to true
}
})
.fail(function(jqXHR, ajaxOptions, thrownError)
{
alert('server not responding...');
});
}
</script>
</body>
</html>
We are include 2 files in index.php page, homedata.php which used to display data in front page and other one we call it inside AJAX calls. ajax-checker.php which used to get data and append after post. So we pass last id from post and get response from ajax-checker.php file.
Also Read : How to import large CSV file in MySQL millions of records.
Create homedata.php file
<?php while($ft=mysqli_fetch_array($row)){ ?>
<div class="col-lg-12 col-sm-12 col-md-12 post-id" id="<?php echo $ft['id']; ?>">
<h4 class="title_class">
<?php echo $ft['post_title']; ?>
</h4>
</a>
<div class="description">
<?php echo html_entity_decode($ft['post_desc']); ?>
</div>
</div>
<?php } ?>
Create ajax-checker.php file
<?php
if ( $_SERVER['REQUEST_METHOD']=='GET' && realpath(__FILE__) == realpath( $_SERVER['SCRIPT_FILENAME'] ) ) {
header( 'HTTP/1.0 403 Forbidden', TRUE, 403 );
exit;
}
require('./config.php');
if(isset($_POST['last_id_sp'])){
$orderby='ASC';
$orderby1="ORDER BY id $orderby";
$sql="SELECT * FROM infinite_scoll WHERE id <'".$_POST['last_id_sp']."' $orderby1 LIMIT 10";
$row = mysqli_query($conn,$sql);
$num=mysqli_num_rows($row);
if($num>=1){
$json = include('homedata.php');
}else{
$json =1;
echo $json;
}
}
?>
Final Output
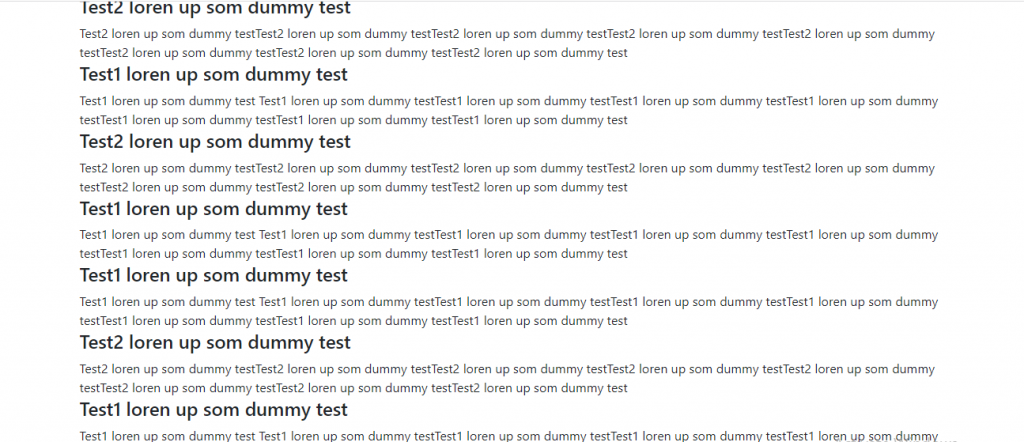