This tutorial we are doing to implement captcha image using PHP GD library. Which helps for spam sending it will be use for Contact form, Registration form etc..
Create index.php file
Create index.php file on root of the project and past below code.
<?php session_start(); ?>
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" crossorigin="anonymous">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap-theme.min.css" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<title>Generate captcha image in php</title>
<body>
<?php
$submit=0;
if(isset($_POST['submit'])){
if($_SESSION['captcha_string']==$_POST["input"])
{
$msg= "Captcha Code is correct. You can proceed";
}
else
{
$msg= "Invalid Captcha Code.";
}
unset($_SESSION['captcha_string']);
$submit=1;
}
$image = imagecreatetruecolor(150, 50);
$background_color = imagecolorallocate($image, 255, 255, 255);
imagefilledrectangle($image,0,0,150,50,$background_color);
$line_color = imagecolorallocate($image, 64,64,64);
$pixel = imagecolorallocate($image, 0,0,255);
for($i=0;$i<500;$i++)
{
imagesetpixel($image,rand()%150,rand()%50,$pixel);
}
$allowed_letters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
$length = strlen($allowed_letters);
$letter = $allowed_letters[rand(0, $length-1)];
$word='';
$text_color = imagecolorallocate($image, 0,0,0);
$cap_length=4;// No. of character in image
for ($i = 0; $i< $cap_length;$i++)
{
$letter = $allowed_letters[rand(0, $length-1)];
imagestring($image, 5, 5+($i*30), 20, $letter, $text_color);
$word.=$letter;
}
$_SESSION['captcha_string'] = $word;
imagepng($image, "captcha_image.png");
?>
<div class="container">
<div class="row">
<div class="col-md-12">
<div class="panel panel-card ">
<div class="panel-body">
<form method="POST" class="text-center">
<?php if(!empty($msg)){ ?>
<div class="form-group">
<?php echo $msg; ?>
</div>
<?php } ?>
<div class="form-group">
<img src="captcha_image.png">
</div>
<div class="form-group">
<input type="text" name="input"/>
</div>
<div class="form-group">
<button type="submit" name="submit" class="btn btn-primary" value="submit">Submit</button>
</div>
</form>
</div>
</div><!-- End .panel -->
</div><!--end .col-->
</div><!--end .row-->
</div>
</body>
</html>
We use PHP image GD library to create image and store value in session. based on user input we compare it if it’s correct or not. below code is for submit user input.
Read also : How to integrate google reCaptcha in PHP
if(isset($_POST['submit'])){
if($_SESSION['captcha_string']==$_POST["input"])
{
$msg= "Captcha Code is correct. You can proceed";
}
else
{
$msg= "Invalid Captcha Code.";
}
unset($_SESSION['captcha_string']);
$submit=1;
}
Final Output
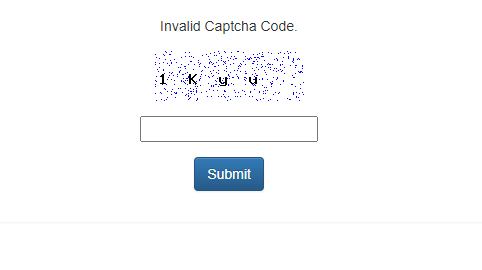