PHP upload file in Dropzone. Dropzone is light weight and easy to use library. You will get it from dropzone official site. Dropzone provide Javascript library which is highly customized and opensource. It is available free to use, user friendly interface and easy to implement it on your existing project.
Previous tutorial we seen upload file in php and We do this similar but this time use dropzone.
let’s have create demo for file upload using dropzone.
Create file index.php for upload file in Dropzone
<!DOCTYPE html> <html> <head> <title>PHP Dropzone File Upload Example Tutorial</title> <link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet"> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <link href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/4.0.1/min/dropzone.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/4.2.0/min/dropzone.min.js"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-md-12"> <h2>PHP Dropzone File Upload on Button Click Example</h2> <form action="upload.php" enctype="multipart/form-data" class="dropzone" id="image-upload"> </form> <button id="uploadFile">Upload Files</button> </div> </div> </div> <script type="text/javascript"> Dropzone.autoDiscover = false; var myDropzone = new Dropzone(".dropzone", { autoProcessQueue: false, maxFilesize: 1, addRemoveLinks: true, acceptedFiles: ".jpeg,.jpg,.png,.gif" }); $('#uploadFile').click(function(){ myDropzone.processQueue(); }); </script> </body> </html>
- autoProcessQueue you can set false or true, true means image upload instantly without click on submit button.
- maxFilesize is set 1 which allow image to upload 1MB increase or decrease as per your needs.
- addRemoveLinks is set true or false based on needs. Remove appear on image when it true so when you will require to delete image from server set it true
- acceptedFiles checks the file’s mime type or extension when you upload the file. We define .jpeg,.jpg,.png,.gif. You will change it based on your needs.
Now create File Upload Code for PHP to accept files and save to server
upload.php
<?php $uploadDir = 'uploads'; if (!empty($_FILES)) { $tmpFile = $_FILES['file']['tmp_name']; $filename = $uploadDir.'/'.time().'-'. $_FILES['file']['name']; move_uploaded_file($tmpFile,$filename); } ?>
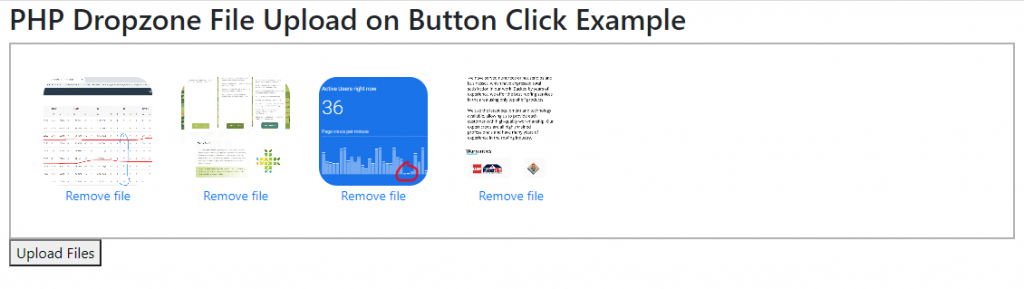
Conclusion
We can create dropdown where you can drag and drop file inside dropzone and upload file. We use similar concept as file upload in php.