What is a REST API?
This tutorial we’ll explain What is REST API is, what is important, how it’s works and how PHP developers can build one effectively.
API stance for Application programming interface, it’s use for communicating between two software program. it usefully for developing web apps, build apps, Mobile Apps Request and Operating System. The Google Maps, Twitter and Facebook API are likely examples.
We will use PHP for developing APIs, I like personally cause of it’s ease of use, support multiple Database integrations, RESTful API Support like (GET, POST, PUT, DELETE) and Many other reasons.
Restful architecture allow data to exchanges over HTTP Request using JSON format.
let’s understand the concepts of stateless communication and client-server model.
stateless communication not storing any data request while client-server model client can request for any data and server give them response back.
Key Components of a REST API
Every REST API consists of endpoints that handle different HTTP requests like GET, POST, PUT, DELETE. Let’s understand bit more in below table.
HTTP Methods | API endpoints | Use |
GET | /user | Fetch All data from User |
POST | /user | Create New User |
PUT | /user/1 | Update user detail by ID |
GET | /user/1 | Retrieve specific user By ID |
DELETE | /user/1 | Delete User By ID |
How REST APIs Work in PHP
- Setting Up PHP for API development
If you are localhost install XAMPP and create project directory called API development or if you are on live create directory structure as below:
- Understanding MySQL Database and JSON response format.
REST APIs send data back as JSON response, it’s lightweight and easy to use.
{ "id": 1, "name": "John", "email": "[email protected]", "password" :"12345" }
- Simple REST API request
Below example, create simple REST API where simply display welcome message using GET Request.
header("Content-Type: application/json"); echo json_encode(["message" => "Welcome to my REST API"]);
Example: Creating a Simple REST API in PHP
Let’s start building simple REST API using PHP that retrieves data from a MySQL database and return data as JSON format.
Create MySQL Table users
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100) NOT NULL, email VARCHAR(100) NOT NULL UNIQUE, password VARCHAR(100) NOT NULL );
Create Connection config.php file
<?php $host = "localhost"; $db_name = "new_programmerdesk"; $username = "root"; $password = ""; $conn = new mysqli($host, $username, $password, $db_name); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); }
Create simple rest api file api.php
<?php header("Content-Type: application/json"); require 'config.php'; /** * @method GET, POST, PUT, DELETE * GET => READ, POST=>INSERT, PUT=>UPDATE, DELETE=>DELETE * file_get_contents("php://input"), true) Format data by ROW */ $method = $_SERVER['REQUEST_METHOD']; $request = $_GET['request'] ?? ''; switch ($method) { case 'GET': $user=!empty($request) ? " WHERE id='".$request."'" : ""; $sql = "SELECT * FROM users ".$user; $result = $conn->query($sql); $users = []; while ($row = $result->fetch_assoc()) { $users[] = $row; } echo json_encode($users); break; case 'POST': $data = json_decode(file_get_contents("php://input"), true); if (!empty($data['name']) && !empty($data['email'])) { $stmt = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)"); $stmt->bind_param("ss", $data['name'], $data['email']); $stmt->execute(); echo json_encode(["message" => "User created successfully"]); } else { echo json_encode(["error" => "Missing name or email"]); } break; case 'PUT': $data = json_decode(file_get_contents("php://input"), true); if (!empty($request) && !empty($data['name']) && !empty($data['email'])) { $stmt = $conn->prepare("UPDATE users SET name=?, email=? WHERE id=?"); $stmt->bind_param("ssi", $data['name'], $data['email'], $request); $stmt->execute(); echo json_encode(["message" => "User updated successfully"]); } else { echo json_encode(["error" => "Missing required parameters"]); } break; case 'DELETE': $data = json_decode(file_get_contents("php://input"), true); if (!empty($request)) { $stmt = $conn->prepare("DELETE FROM users WHERE id=?"); $stmt->bind_param("i", $request); $stmt->execute(); echo json_encode(["message" => "User deleted successfully"]); } else { echo json_encode(["error" => "Missing user ID"]); } break; default: echo json_encode(["error" => "Invalid request method"]); } $conn->close(); ?>
Create .htaccess file for user-friendly slug
RewriteEngine On # Turn on the rewriting engine RewriteRule ^user$ api.php [NC,L] RewriteRule ^user/([0-9_-]*)$ api.php?request=$1 [NC,L]
Test REST API using Postman.
- READ : GET (Retrieve all user table data)
curl -X GET http://localhost/user
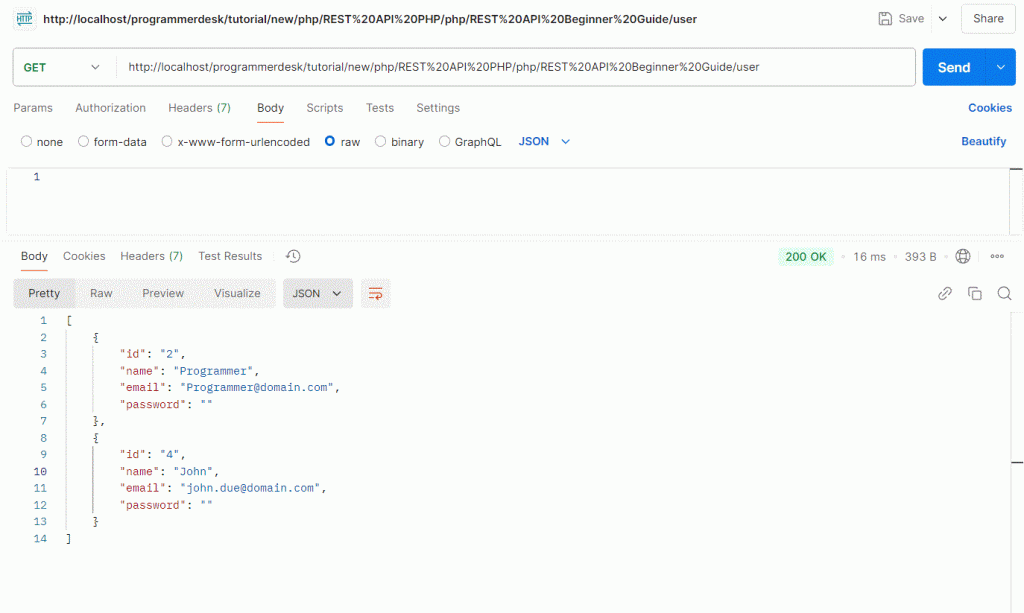
- READ : GET (Retrieve single value)
curl -X GET http://localhost/user/{$id}
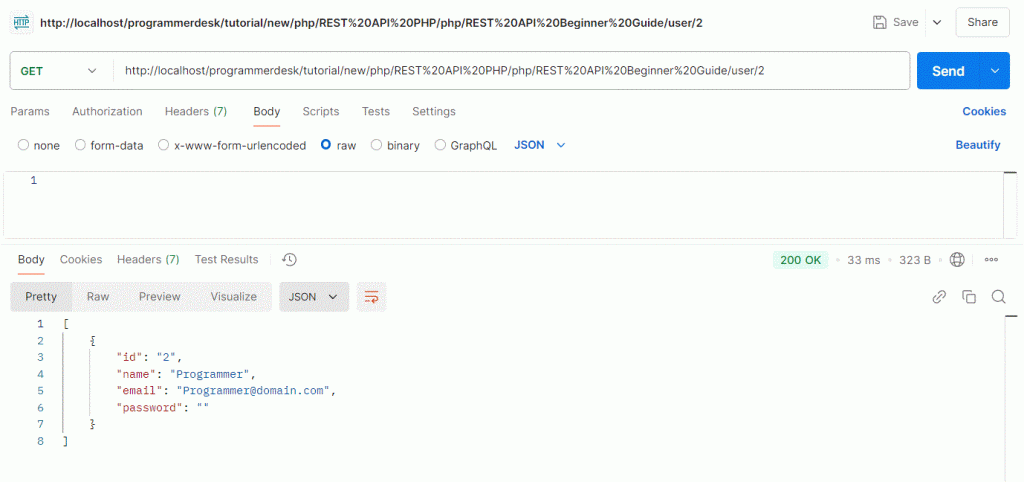
- INSERT : POST(Add data in table)
curl -X POST http://localhost/user
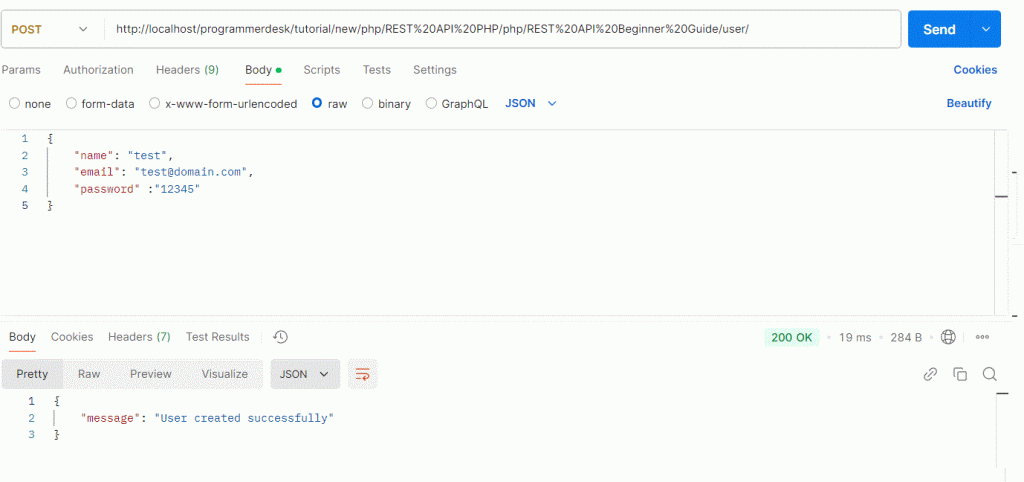
- Update User : PUT
curl -X POST http://localhost/user/{$id}
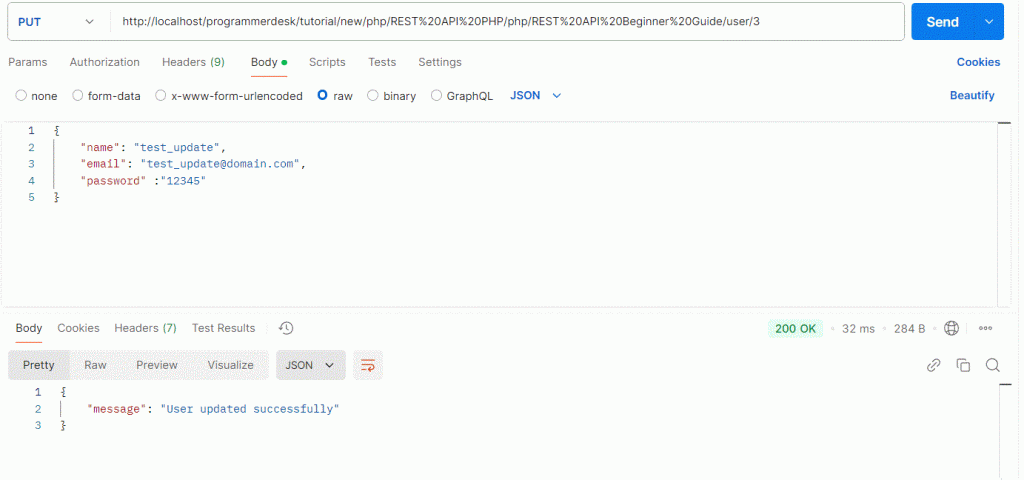
- Delete User : DELETE
curl -X DELETE http://localhost/user/{$id}
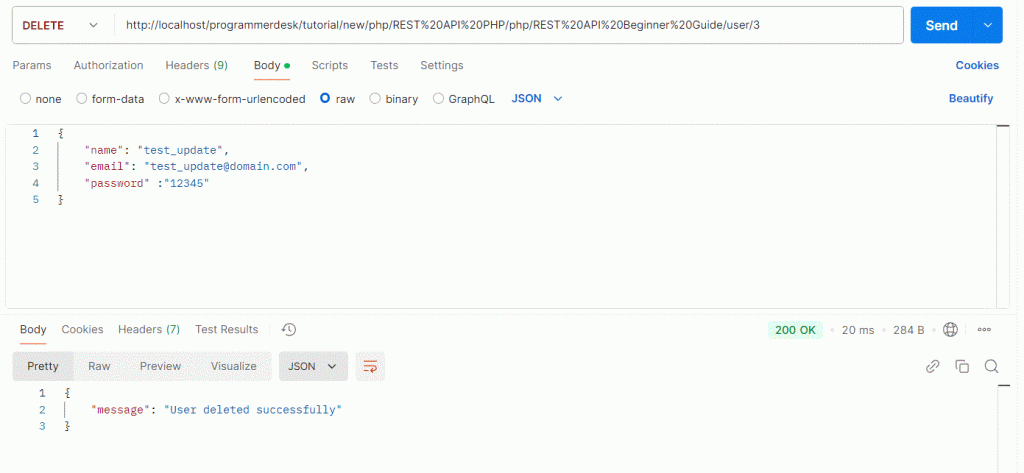
Best Practices for Building REST APIs in PHP
Build secure REST API in PHP to use JSON response,
For developing advance level of security, use JWT Authentications or OAuth2. Those mostly use of developing mobile apps request API.
Add validations and implement rate limiting.
Implement Error handling and Optimized Database query for better Performance.
FAQ
What is rest API in PHP?
A Rest API allow developers to exchange data though GET, PUT, POST, DELETE HTTP method using JSON Response.
How do I create simple REST API?
You can create simple rest API by using HTTP Method, Setting up Router and Add Headers. Here is simple example.
header("Content-Type: application/json"); echo json_encode(["message" => "Welcome to my REST API"]);
Conclusion
In this tutorial, we covered :
- What is REST APIs, why that important.
- Key Components of a REST API like GET, POST, PUT, DELETE
- Create Simple REST API using MySQL Database.