google recaptcha integratein PHP, here are steps to follow:
- Go to https://www.google.com/recaptcha/admin/create
- Enter domain name and select reCaptcha type.
- Generate site and secret key.
- Create file and add google script
<script src='https://www.google.com/recaptcha/api.js' async defer ></script>
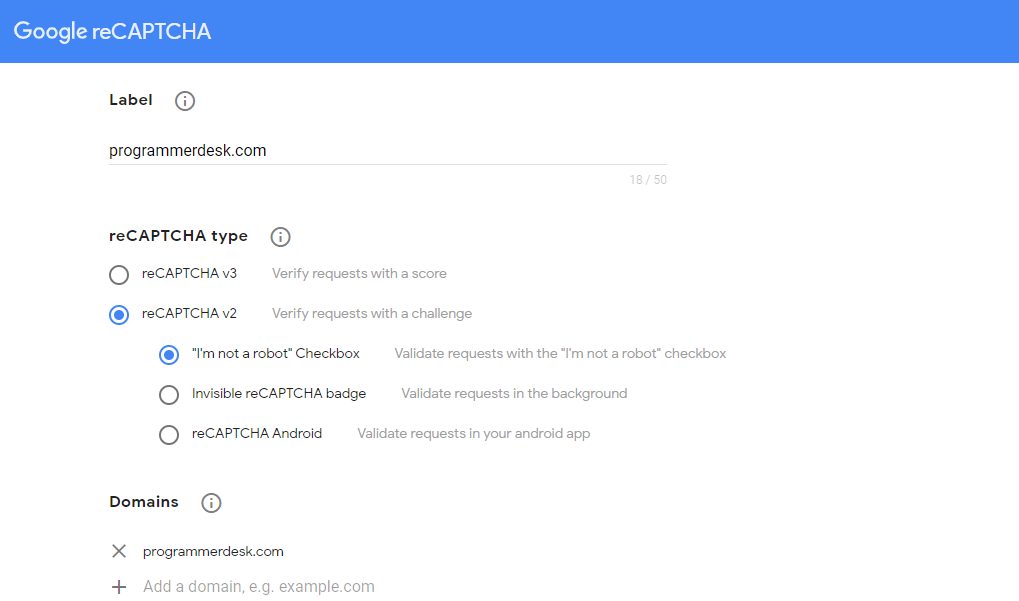
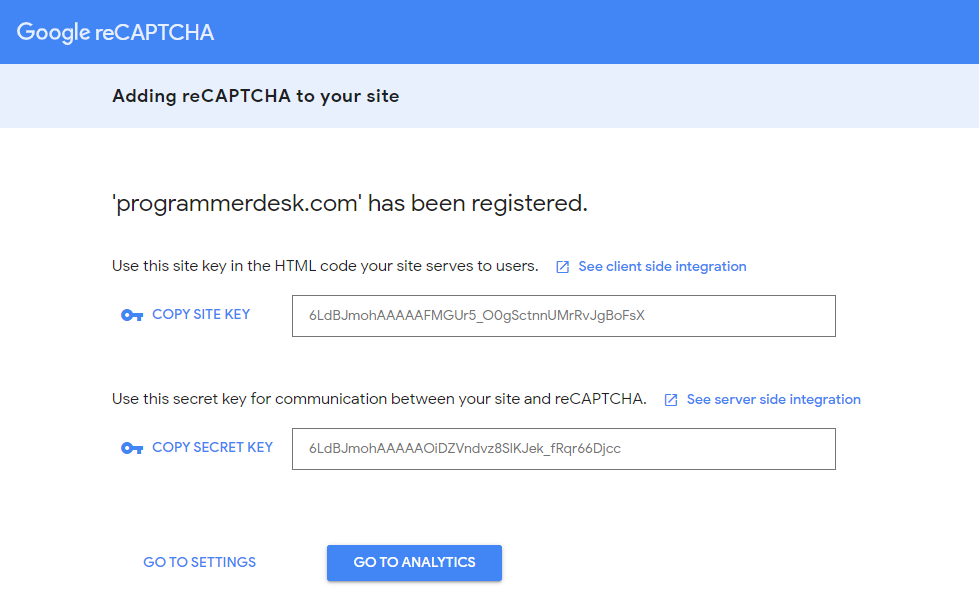
Create index.php file
<html>
<head>
<script src='https://www.google.com/recaptcha/api.js' async defer ></script>
</head>
<body>
<form action="verify.php" method="post">
<div class="g-recaptcha" data-sitekey="ENTER_SITE_KEY_HERE"></div>
<input type="submit" name="submit" value="SUBMIT">
</form>
</body>
</html>
Add site key inside file and create new file call verify.php. you can use in any form eg. signup, signing, contact etc..
Read Also: How to generate captcha image in php
Create verify.php file
<?php
if(!empty($_POST['g-recaptcha-response']))
{
$secret = 'SECRET_KEY_HERE';
$verifyResponse = file_get_contents('https://www.google.com/recaptcha/api/siteverify?secret='.$secret.'&response='.$_POST['g-recaptcha-response']);
$responseData = json_decode($verifyResponse);
if($responseData->success)
$message = "Captcha varification successfully";
else
$message = "Captcha varification fail.";
echo $message;
}
?>
It will generate response in JSON format you can decode it. return success or fail response.