This tutorial we are doing to implement captcha image in PHP using PHP GD library. Captcha helps for automation submission of form like Registrations, Contact Us or Custom Forms. by adding captcha reducing spam bots and enhancing securing.
Why Use CAPTCHA in PHP?
- Reducing spam submission.
- Auto bot submission of button click.
- Enhance the security.
- Easy to use and implement using PHP GD library.
Create Captcha image in PHP
Create class captcha.php and generate random captcha image.
<?php class captcha{ public $width; public $height; public function __construct($width,$height){ session_start(); $this->width=$width; $this->height=$height; } public function createCaptcha(){ // Generate a random CAPTCHA string $captcha_code = substr(str_shuffle('ABCDEFGHJKLMNPQRSTUVWXYZ23456789'), 0, 5); $_SESSION['captcha'] = $captcha_code; // Create CAPTCHA image $image = imagecreatetruecolor($this->width, $this->height); // Colors $background_color = imagecolorallocate($image, 255, 255, 255); $text_color = imagecolorallocate($image, 0, 0, 0); $line_color = imagecolorallocate($image, 64, 64, 64); $dot_color = imagecolorallocate($image, 100, 100, 100); // Fill background imagefilledrectangle($image, 0, 0, $this->width, $this->height, $background_color); // Add noise (dots) for ($i = 0; $i < 500; $i++) { imagesetpixel($image, rand(0, $this->width), rand(0, $this->height), $dot_color); } // Add noise (lines) for ($i = 0; $i < 5; $i++) { imageline($image, rand(0, $this->width), rand(0, $this->height), rand(0, $this->width), rand(0, $this->height), $line_color); } // Add text to image $font = __DIR__ . '/Arial.ttf'; // Make sure you have this TTF font file in the same directory imagettftext($image, 20, rand(-10, 10), 20, 35, $text_color, $font, $captcha_code); // Output image header('Content-Type: image/png'); imagepng($image); imagedestroy($image); } } $ca=new captcha(150,50); $ca->createCaptcha(); ?>
How it works :
Initialize captcha class with pass width and height. It can be generate five random character each time and ensure you have added Arial.ttf font in directory file.
Display Captcha in HTML Form.
Create form.php file and add captcha class for displaying image. This will create captcha image with input field for user to enter. Clicking on Refresh captcha button reloads the captcha.php file and Date.now() this will helps for prevent catching.
Read also : How to integrate google reCaptcha in PHP
<?php session_start(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { if (!empty($_POST['captcha_input']) && $_POST['captcha_input'] == $_SESSION['captcha']) { echo "✅ Form submitted successfully."; } else { echo "❌ Incorrect CAPTCHA. Please try again."; } } ?> <form action="" method="post"> <label>Enter CAPTCHA:</label><br> <img src="captcha.php" alt="CAPTCHA" id="captchaImage"><br> <button type="button" onclick="refreshCaptcha()">🔄 Refresh CAPTCHA</button><br><br> <input type="text" name="captcha_input" required><br><br> <button type="submit">Submit</button> </form> <script> function refreshCaptcha() { document.getElementById('captchaImage').src = 'captcha.php?' + Date.now(); } </script>
Final Output
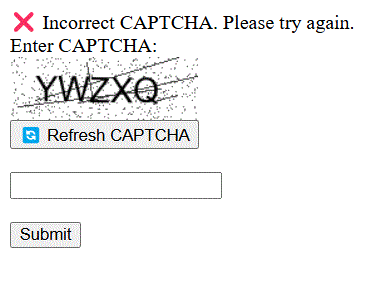
Conclusion.
- Create Random Captcha image in PHP
- Display the captcha inside form.php file.
- Refresh button for Captcha
- Form submission with correct captcha input.
- Adding CAPTCHA it’s prevents spam and improve security