In this tutorial we are going to create contact us form using PHP and jQuery. Contact Us form are always needed on website to contact website owner.
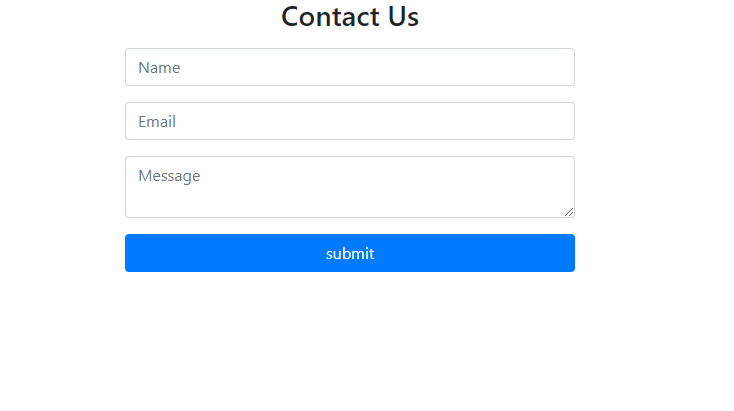
Create HTML contact us form
<!DOCTYPE html>
<html>
<head>
<title>How to develop Contact Us form using PHP and jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.19.1/jquery.validate.min.js"></script>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" />
</head>
<body class="container">
<div class="row">
<div class="account-col" style="width: 450px;text-align: center;margin: 0px auto;">
<h3>Contact Us</h3>
<?php if($msg!=1) {
echo $msg;
} ?>
<form class="m-t" role="form" method="post">
<div class="form-group">
<span style="color: #b70a0a;"></span>
</div>
<div class="form-group">
<input type="text" class="form-control" name="name" placeholder="Name" required="">
</div>
<div class="form-group">
<input type="text" class="form-control" name="email" placeholder="Email">
</div>
<div class="form-group">
<textarea class="form-control" name="message" placeholder="Message"></textarea>
</div>
<input type="submit" class="btn btn-primary btn-block" name="submit" value="submit">
</form>
</div>
</div>
</body>
</html>
On submit form you can add action or add below code to top of the page. I prefer to add it on top of the HTML file.
<?php
$toEmail="admin@domain.com";
$msg=1;
$sendemail=0;
if(isset($_POST['submit'])){
if(!empty($_POST['name']) && !empty($_POST['email']) && !empty($_POST['message'])){
if(filter_var($_POST['email'], FILTER_VALIDATE_EMAIL) === false){
$msg="Please enter valid email address.";
}else{
$subject = 'Contact Request from '.$_POST['name'];
$body = '<h2>Contact Request</h2>
<h4>Name</h4><p>'.$_POST['name'].'</p>
<h4>Email</h4><p>'.$_POST['email'].'</p>
<h4>Message</h4><p>'.$_POST['message'].'</p>';
// Set content-type header for sending HTML email
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
// Additional headers
$headers .= 'From: '.$_POST['name'].'<'.$_POST['email'].'>'. "\r\n";
// Send email
$send = mail($toEmail, $subject, $body, $headers);
if($send){
$sendemail=1;
$msg = 'Your contact request has been submitted successfully.';
}else{
$msg = 'Your contact request submission failed, please try again.';
}
}
}else{
$msg="Please fill all the details.";
}
}
?>
You can change $toEmail=”admin@domain.com”; as per your requirements.
I hope you like this tutorial