In this tutorial we are going to learn Convert HTML to PDF and use jsPDF, html2canvas and window.print(). this will help with generating invoices, receipt, Reports and Web Content print. As we already implement Convert HTML to PDF using DOMPdf, that support PHP and HTML for generating PDF. Now jsPDF is pure JS lib
jsPDF lib provide all customized solutions and we can try to covered all the require scenario, In snippet we explain jsPDF lib with detail description and demo.
Method 1: Generate Simple Text PDF Using jsPDF
- Step 1: Include jsPDF
include jspdf cdn on top of the file.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/2.5.1/jspdf.umd.min.js"></script>
- Step 2: Create a Download Button
<button id="download">Download PDF</button> <script> document.getElementById('download').addEventListener('click', function() { const { jsPDF } = window.jspdf; const doc = new jsPDF(); doc.setFont("times"); doc.setFontSize(16); doc.text("Hello, this is a PDF!", 10, 10); doc.text("Generated using jsPDF.", 10, 20); doc.save("simple.pdf"); }); </script>
on download button click, initiase jsPDF() class of jsPDF lib.
doc.setFont : Set font (courier, times, helvetica) as in-build
doc.setFontSize : Set text fontsize, in out case 16
doc.text : Set text with top and right padding.
doc.save : Save PDF file.
Method 2: Use html2canvas for Convert HTML to PDF
- Step1: Include html2canvas CDN
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/1.4.1/html2canvas.min.js"></script>
- Step2 : Add download button and html2canvas script
<div id="content" style="padding: 20px; border: 1px solid #ddd; background: #f9f9f9;"> <h1 style="color:red;background:yello;">Invoice #001</h1> <p>Customer: John Doe</p> <p>Date: <span id="date"><?php echo date("d/m/Y"); ?></span></p> <p>Loren ipsum d ummy yect text a loren dummy text loren ipsum text dummy text, </p> <table border="0" style="width: 100%;text-align: left;" id="invoiceTable"> <tr> <th>Item</th> <th>Price</th> </tr> <tr> <td>Product A</td> <td>$50</td> </tr> <tr> <td>Product B</td> <td>$30</td> </tr> <tr> <td>Product C</td> <td>$50</td> </tr> <tr> <td>Product D</td> <td>$30</td> </tr> </table> </div> <button id="download">Download PDF</button> <script> document.getElementById('download').addEventListener('click', function() { const { jsPDF } = window.jspdf; const doc = new jsPDF(); html2canvas(document.querySelector("#content")).then(canvas => { const imgData = canvas.toDataURL('image/png'); const imgWidth = 190; const imgHeight = (canvas.height * imgWidth) / canvas.width; doc.addImage(imgData, 'PNG', 10, 10, imgWidth, imgHeight); doc.save("invoice.pdf"); }); }); </script>
Onclick download button initialize jspdf lib and it will generate pdf automatically with preserves HTML styles and images.
doc.addImage(imgData, 'PNG', 10, 10, imgWidth, imgHeight) 10, 10 : Start from top left corner of page
imgWidth :keep image inside PDF width. A4 page width is 210mm and we keep this 190
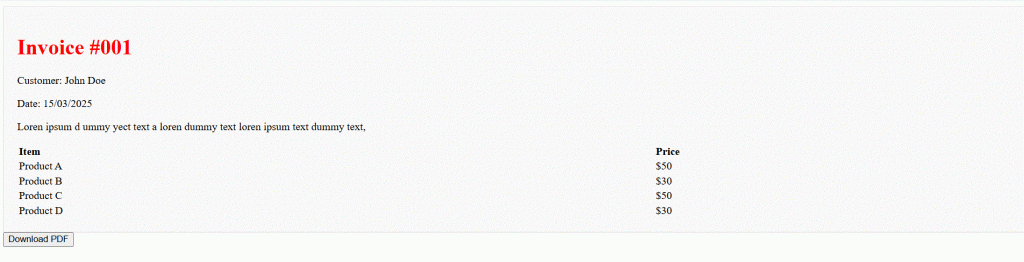
Method 3: Use html2canvas for Convert HTML to PDF
- Step1 : Call jspdf autotable lib on head section.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf-autotable/3.5.23/jspdf.plugin.autotable.min.js"></script>
autoTable works for table only it support many attributes with customized parameter settings.
Step2 : Call autoTable class and customized parameter settings.
<script> doc.autoTable({ html: '#invoiceTable', startY: 20, styles: { font: "times", fontSize: 12, textColor: [255, 0, 0] }, // Apply styles inside table headStyles: { fillColor: [0, 102, 204], textColor: [255, 255, 255] }, // Header background blue bodyStyles: { fontStyle: "bold", textColor: [0, 0, 0] }, // Make text black alternateRowStyles: { fillColor: [240, 240, 240] } // Light grey for alternate rows }); doc.save("invoice.pdf"); </script>
html: ‘#invoiceTable’ HTML table name
startY : Start from top position
styles : apply styles for inside table.
font : jsPDF has support three default fonts: times, courier, helvetica (default)
headStyles : Apply styles for header row.
bodyStyles : Apply styles for table body.
alternateRowStyles : Use for alternate rows style.
Method 4: Multi-Page PDF Handling
if page content is longer and not able to view on one page. Use addPage() to create multiple pages dynamically.
- We use html2canvas for capture data and convert into image using toDataURL
- Check imgHeight if more than 250 create new page using doc.addPage() and then Add Remaining part on second page.
<script> const doc = new jsPDF(); let y = 10; // Starting position html2canvas(document.querySelector("#content")).then(canvas => { const imgData = canvas.toDataURL('image/png'); let imgHeight = (canvas.height * 190) / canvas.width; // If image is too tall, split it across pages if (imgHeight > 250) { doc.addImage(imgData, 'PNG', 10, y, 190, 250); doc.addPage(); // Create a new page doc.addImage(imgData, 'PNG', 10, 10, 190, imgHeight - 250); } else { doc.addImage(imgData, 'PNG', 10, y, 190, imgHeight); } doc.save("multipage.pdf"); }); </script>