This tutorial we are going to implement custom drag and drop builder for image upload using JS. We will use bootstrap for designing and JS library to control drag and drop builder. So let’s have begin with create HTML.
Create index.html file.
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" crossorigin="anonymous"> </head> <body> <div class="container"> <div class="row"> <div class="col-md-12"> <div class="panel panel-card "> <div class="panel-body"> <div class="form-group mb-10"> <div class="drag-area"> <div class="icon"> <svg xmlns="http://www.w3.org/2000/svg" width="55" height="55" fill="currentColor" class="bi bi-upload" viewBox="0 0 16 16"> <path d="M.5 9.9a.5.5 0 0 1 .5.5v2.5a1 1 0 0 0 1 1h12a1 1 0 0 0 1-1v-2.5a.5.5 0 0 1 1 0v2.5a2 2 0 0 1-2 2H2a2 2 0 0 1-2-2v-2.5a.5.5 0 0 1 .5-.5z"></path> <path d="M7.646 1.146a.5.5 0 0 1 .708 0l3 3a.5.5 0 0 1-.708.708L8.5 2.707V11.5a.5.5 0 0 1-1 0V2.707L5.354 4.854a.5.5 0 1 1-.708-.708l3-3z"></path> </svg> </div> <header>Drag & Drop to Upload File</header> <span>OR</span> <button class="btn-theme">Browse File</button> <input type="file" hidden accept=".jpg, .jpeg, .png" name="file_logo" id="file_logo"> </div> <p class="logo_error" style="display:none;">Your logo dimensions are not valid. The logo should be 400px width by 200px height.</p> <p class="help">Dimensions must be 400px width by 200px height <br>(JPEG or PNG format) <br></p> </div> </div> </div> <!-- End .panel --> </div> <!--end .col--> </div> <!--end .row--> </div> <script src="jquery-main.js"></script> </body> </html>
We have ready with form now, we will add some styles in drag and drop builder to looks awesome. so, let’s create the style.css in working directory and paste this code.
Create style.css
#file_logo{display:none;} .drag-area { border: 2px dashed #2a232357; width: 100%; align-items: center; justify-content: center; flex-direction: column; text-align: center; max-width:400px; height:200px; } .drag-area .icon { font-size: 70px; } .drag-area header { font-weight: 500; } .drag-area span { font-size: 25px; font-weight: 500; margin: 10px 0 15px 0; } .btn-theme { background-color: #D61F8B; border: 1px solid #D61F8B; border-radius: 5px; color: #fff; display: inline-block; font-size: 15px; padding: 8px 35px 8px; text-align: center; }
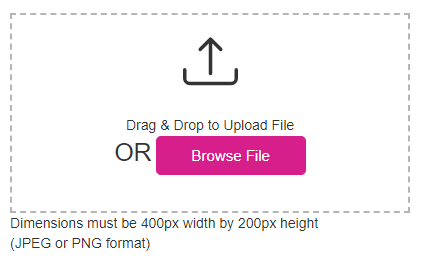
Now, We are ready with image upload drag and drop builder. Let’s create JS file and add this on builder to make it working. create another JS file dragdrop.js and include in index.html file.
Create dragdrop.js file
const dropArea = document.querySelector(".drag-area"), dragText = dropArea.querySelector("header"), button = dropArea.querySelector("button"), input = dropArea.querySelector("input"); let file; //this is a global variable and we'll use it inside multiple functions dropArea.onclick = ()=>{ input.click(); //if user click on the button then the input also clicked } input.addEventListener("change", function(){ //getting user select file and [0] this means if user select multiple files then we'll select only the first one file = this.files[0]; dropArea.classList.add("active"); showFileLogo(); //calling function }); //If user Drag File Over DropArea dropArea.addEventListener("dragover", (event)=>{ event.preventDefault(); //preventing from default behaviour dropArea.classList.add("active"); dragText.textContent = "Release to Upload File"; }); //If user leave dragged File from DropArea dropArea.addEventListener("dragleave", ()=>{ dropArea.classList.remove("active"); dragText.textContent = "Drag & Drop to Upload File"; }); //If user drop File on DropArea dropArea.addEventListener("drop", (event)=>{ event.preventDefault(); //preventing from default behaviour //getting user select file and [0] this means if user select multiple files then we'll select only the first one file = event.dataTransfer.files[0]; showFileLogo(); //calling function }); function showFileLogo(){ let fileType = file.type; //getting selected file type let validExtensions = ["image/jpeg", "image/jpg", "image/png"]; //adding some valid image extensions in array if(validExtensions.includes(fileType)){ //if user selected file is an image file let fileReader = new FileReader(); //creating new FileReader object fileReader.onload = ()=>{ let fileURL = fileReader.result; //passing user file source in fileURL variable let imgTag = `<img src="${fileURL}" alt="">`; //creating an img tag and passing user selected file source inside src attribute var i = new Image(); i.onload = function(){ var width= i.width; var height= i.height; if(width==400 && height==200){ dropArea.innerHTML = imgTag; //adding that created img tag inside dropArea container }else{ $(".logo_error").show().fadeOut(2800) } }; i.src = fileURL; } fileReader.readAsDataURL(file); }else{ alert("This is not an Image File!"); dropArea.classList.remove("active"); dragText.textContent = "Drag & Drop to Upload File"; } }
Read Also: How to compress image size while uploading in PHP
When you can upload image on drag and drop div it will call file browse button.
We have applied some restriction for file upload :
- Accept PNG and JPEG only
- Image size should be 400 *200 change it as per your needs.
- Image view on drag and drop div using fileReader.
- alert message when anything goes wrong.