This tutorial we are going to learn submit form without page refresh using PHP, jQuery and AJAX. We will insert data in table when user input the value on form submit.
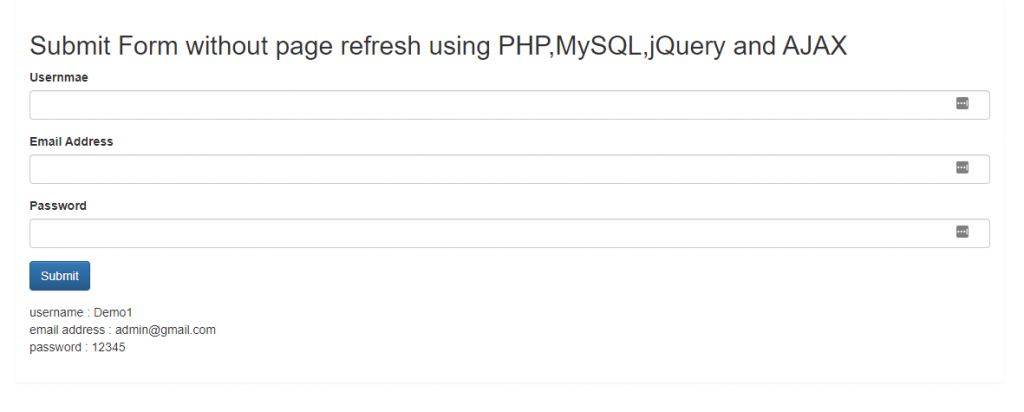
Create MySQL table
<?php $servername = "localhost"; $username = "root"; $password = ""; $db = "test"; $conn = mysqli_connect($servername, $username, $password, $db); // Check The Connection if (mysqli_connect_errno() ){ echo "Database Connection Failed: " . mysqli_connect_error(); die; } mysqli_set_charset($conn,"utf8"); ?>
Table created after design HTML form. We will use bootstrap for this.
Create index.php file
<?php include('config.php'); ?> <!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" crossorigin="anonymous"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap-theme.min.css" crossorigin="anonymous"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-md-12"> <div class="panel panel-card "> <div class="panel-body"> <h2>Submit Form without page refresh using PHP,MySQL,jQuery and AJAX</h2> <form method="post" id="form_data"> <div class="form-group"><label>Usernmae</label> <input type="text" class="form-control" name="username"> </div> <div class="form-group"><label>Email Address</label> <input type="text" class="form-control" name="email_address"> </div> <div class="form-group"><label>Password</label> <input type="text" class="form-control" name="password"> </div> <div class="form-group"> <button type="submit" class="btn btn-primary" id="submit_frm" value="Submit">Submit</button> </div> <div class="form-group result"> </div> </form> </div> </div> <!-- End .panel --> </div> <!--end .col--> </div> <!--end .row--> </div> <script> $(document).ready(function () { $('#submit_frm').click(function (e) { e.preventDefault(); var username=$("input[name=username]").val(); var email_address=$("input[name=email_address]").val(); var password=$("input[name=password]").val(); $.ajax ({ type: "POST", url: "ajax_request.php", data: { "username": username, "email_address": email_address, "password": password }, success: function (data) { $('.result').html(data); $('#form_data')[0].reset(); } }); }); }); </script> </body> </html>
ajax_request.php
file we will get post data and process in database.
Create ajax_request.
php file
<?php ## Database configuration include 'config.php'; //insert into database if(!empty($_POST['username']) && !empty($_POST['email_address']) && !empty($_POST['password'])) { $username = $_POST['username']; $email_address = $_POST['email_address']; $password = $_POST['password']; if(mysqli_query($conn, "insert into users (username, email_address, password) values ('$username', '$email_address', '$password')")){ echo "username : ".$username."</br>"; echo "email address : ".$email_address."</br>"; echo "password : ".$password."</br>"; }else{ echo "Data not inserted correctly."; } }
Also Read: asdasa