In this tutorial we are going to learn Image compression in PHP. Optimized image is most use for enhancing speeds, quick loading and helps for improving SEO ranking. Image compression helps you to reduce image so, if user upload large image we can reduce size of image then upload. It is good idea to optimized size before upload and store in DB. In this tutorial we can use GD library in our example.
Why Image Compression is Important?
- Improve website loading time, which directly effected with SEO.
- lower bounce rate, if website loading time is longer visitor may leave site quickly which directly effected with bounce rate.
- Improve core web vitals, if all images are compressed which helps for LCP score
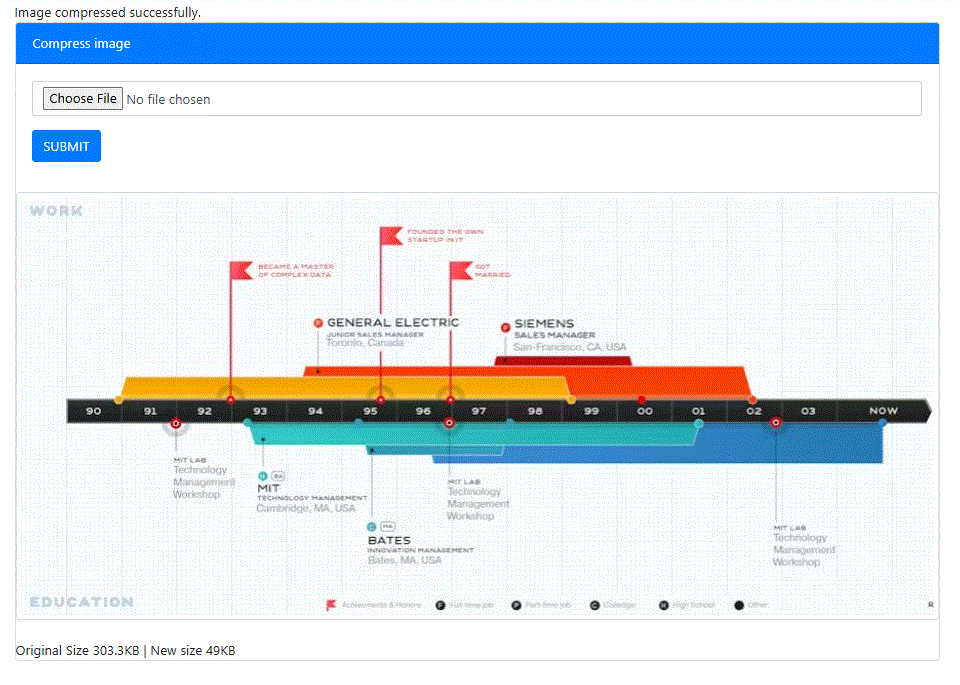
Create DB table
CREATE TABLE images ( id INT AUTO_INCREMENT PRIMARY KEY, filename VARCHAR(255) NOT NULL );
Create DB Connection file (db.php)
<?php $host = "127.0.0.1:3360"; $db_name = "new_programmerdesk"; $username = "root"; $password = ""; $conn = new mysqli($host, $username, $password, $db_name); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); }
Create HTML file upload form
Make sure HTML form should be
- method="post"
- enctype="multipart/form-data"
<html> <head> <link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous"> </head> <body> <div class="container"> <div class="row"> <div class="col"> <?php echo isset($statusmsg)? $statusmsg : ""; ?> <div class="card"> <div class="card-header bg-primary text-white">Compress image </div> <div class="card-body"> <form action="" method="post" enctype="multipart/form-data"> <div class="form-group"> <input type="file" class="form-control" name="image" id="image"> </div> <div class="mx-auto"> <input type="submit" name="submit" class="btn btn-primary text-right" value="SUBMIT"> </div> </form> </div> <?php if($status == 'success'){ echo "<img src='".$image_path."' class='img-thumbnail'><br/>"; echo "Original Size ".convertToReadableSize($imagesize) ." | New size ".$getsize."<br/>"; } ?> </div> </div> </div> </div> </body> </html>
Best Methods for Image Compression in PHP
GD library is effective and easy to use, which include in PHP by default. Below function we use for compress image if it’s JPG, PNG or GIF type extension.
function compressImage($source, $destination, $quality) { // Get image info $imgInfo = getimagesize($source); $mime = $imgInfo['mime']; // Create a new image from file switch($mime){ case 'image/jpeg': $image = imagecreatefromjpeg($source); break; case 'image/png': $image = imagecreatefrompng($source); break; case 'image/gif': $image = imagecreatefromgif($source); break; default: $image = imagecreatefromjpeg($source); } // Save image $response=imagejpeg($image, $destination, $quality); // Return compressed image return $response; }
- $source pass file name that compress image size.
- $destination store compress image to that directory.
- $quality Image quality which set from 60 to 70 for better image result quality.
There are also other way for compressing images using Imagick and TinyPNG API but in our example we can create demo of GD Library.
Convert Image to readable Format size.
function convertToReadableSize($size){ $base = log($size) / log(1024); $suffix = array("", "KB", "MB", "GB", "TB"); $f_base = floor($base); return round(pow(1024, $base - floor($base)), 1) . $suffix[$f_base]; }
Uploading, Compressing, and Storing an Image with GD
On form submit button store image and compress image size by calling compressImage() this function.
// File upload path $upload_dir = "images/"; // If file upload form is submitted $status = $statusmsg = $compressedImageSize=$getsize=$image_path=''; if(isset($_POST["submit"])){ $status = 'error'; if(!empty($_FILES["image"]["name"])) { // File info $filename = basename($_FILES["image"]["name"]); $image_path = $upload_dir . $filename; $fileType = pathinfo($image_path, PATHINFO_EXTENSION); // Allow certain file formats $allowtypes = array('jpg','png','jpeg','gif'); if(in_array($fileType, $allowtypes)){ // Image temp source $imagetemp = $_FILES["image"]["tmp_name"]; $imagesize = $_FILES["image"]["size"]; // Compress size and upload image $compressedImage = compressImage($imagetemp, $image_path, 80); if(!empty($compressedImage)){ $stmt = $conn->prepare("INSERT INTO images (filename) VALUES (?)"); $stmt->bind_param("s", $filename); $stmt->execute(); $compressedImageSize = filesize($image_path); $getsize=convertToReadableSize($compressedImageSize); $status = 'success'; $statusmsg = "Image compressed successfully."; }else{ $statusmsg = "Image compress failed!"; } }else{ $statusmsg = 'Sorry, only JPG, JPEG, PNG, & GIF files are allowed to upload.'; } }else{ $statusmsg = 'Please select an image file to upload.'; } }
Conclusion
This examples helps you for compress image without loosing quality, we used inbuild GD library of PHP. compressImage() this functions compress JPEG, JPG, PNG and GIF images.