In this tutorial, we are going to implement forgot password feature in PHP. We already implement mail sending using PHPMailer and login functions. Forgot Password is needed for authentication feature. When user forgot his login password, they simply enter email and this script can generate random token. which sent to user mail inbox and then generate new password.
Now, we can guide you step by step to implement forgot password email functionality.
Step 1: Database Setup
Create database table users, if you have login table then modify it by adding token field.
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, email VARCHAR(255) UNIQUE NOT NULL, password VARCHAR(255) NOT NULL, reset_token VARCHAR(255), token_expiry DATETIME );
Step 2: Install PHPMailer
Install composer and PHPMailer for secure mail transfer.
composer require phpmailer/phpmailer
Step 3: Create a Database Connection File (db.php
)
Here, we defined all constant variable for SMTP. Replace it as per your needed.
<?php $host = "127.0.0.1:3360"; $db_name = "new_programmerdesk"; $username = "root"; $password = ""; //SMTP mail configuration. define('HOST', 'domain.com'); define('USERNAME', '[email protected]'); define('PASSWORD', 'yourpassword'); define('DOMAIN','https://domain.com/forgot-password'); $conn = new mysqli($host, $username, $password, $db_name); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } ?>
Step 3: Create Forgot Password Form (mail.php
)
This form contain form input field and submit button. We use bootstrap for design HTML elements.
<html> <head> <link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous"> </head> <body> <div class="container"> <div class="row"> <div class="col"> <?php echo isset($msg)? $msg : ""; ?> <div class="card"> <div class="card-header bg-primary text-white"><i class="fa fa-envelope"></i> Reset Password </div> <div class="card-body"> <form method="post" action=""> <div class="form-group"> <label for="email">Email address</label> <input type="email" class="form-control" name="email" id="email" aria-describedby="emailHelp" placeholder="Enter email" required> <small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small> </div> <div class="mx-auto"> <input type="submit" name="submit" class="btn btn-primary text-right" value="SUBMIT"> </div> </form> </div> </div> </div> </div> </div> </body> </html>
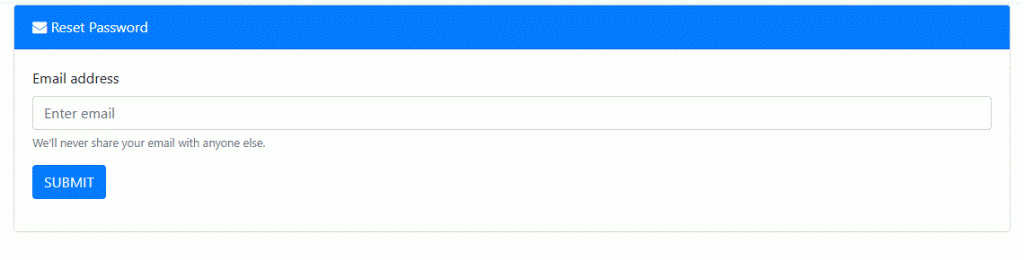
Step 4: Send Reset Link Using PHPMailer
When submit form call POST request and check if email exists on users table. If exists generate token and sent mail using PHPMailer.
<?php require 'db.php'; require './vendor/autoload.php'; use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; if ($_SERVER["REQUEST_METHOD"] == "POST") { $email = $_POST["email"]; $stmt = $conn->prepare("SELECT * FROM users WHERE email = ?"); $stmt->bind_param("s", $email); $stmt->execute(); $result = $stmt->get_result(); $user = $result->fetch_assoc(); if ($user) { $token = bin2hex(random_bytes(20)); $expires = date("Y-m-d H:i:s", strtotime("+1 hour")); $stmt = $conn->prepare("UPDATE users SET reset_token = ?, token_expiry = ? WHERE email = ?"); $stmt->bind_param("sss", $token, $expires, $email); $stmt->execute(); $resetLink = DOMAIN."/reset_password.php?token=$token"; $mail = new PHPMailer(true); try { $mail->isSMTP(); $mail->Host = HOST; // Change to your SMTP provider $mail->SMTPAuth = true; $mail->Username = USERNAME; $mail->Password = PASSWORD; $mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS; $mail->Port = 587; $mail->setFrom('[email protected]', 'No Reply'); $mail->addAddress($email); $mail->isHTML(true); $mail->Subject = "Password Reset"; $mail->Body = "Click the link to reset your password: <a href='$resetLink'>$resetLink</a>"; $mail->send(); $msg = "Check your email for the password reset link."; } catch (Exception $e) { $msg = "Email could not be sent. Mailer Error: {$mail->ErrorInfo}"; } } else { $msg = "Email not found!"; } } ?>
Step 5: Reset Password Page(reset_password.php)
On visit page URL, this page will help for checking if token exists then change password. Before go with PHP logic we can design HTML Page.
<html> <head> <link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous"> </head> <body> <div class="container"> <div class="row"> <div class="col"> <?php echo isset($msg)? $msg : ""; ?> <?php if($error==0) { ?> <div class="card"> <div class="card-header bg-primary text-white"> <i class="fa fa-envelope"></i> Reset Password </div> <div class="card-body"> <form action="update_password.php" method="POST"> <div class="form-group"> <input type="hidden" name="token" value="<?php echo $token; ?>"> <input type="password" class="form-control" name="password" placeholder="Enter new password" required> </div> <div class="mx-auto"> <input type="submit" name="submit" class="btn btn-primary text-right" value="SUBMIT"> </div> </form> </div> </div> <?php } ?> </div> </div> </div> </body> </html>
Check validation token and expiry time using PHP
<?php require 'db.php'; $error=0; if (isset($_GET['token'])) { $token = $_GET['token']; $stmt = $conn->prepare("SELECT * FROM users WHERE reset_token = ? AND token_expiry > NOW()"); $stmt->bind_param("s", $token); $stmt->execute(); $result = $stmt->get_result(); $user = $result->fetch_assoc(); if (!$user) { $error=1; $msg="Invalid or expired token!"; } } else { $error=1; $msg="No token provided!"; }
Step 6: Update Password (update_password.php)
Based on user input update password and removed token.
<?php require 'db.php'; if ($_SERVER["REQUEST_METHOD"] == "POST") { $token = $_POST["token"]; $newPassword = password_hash($_POST["password"], PASSWORD_BCRYPT); $stmt = $conn->prepare("UPDATE users SET password = ?, reset_token = NULL, token_expiry = NULL WHERE reset_token = ?"); $stmt->bind_param("ss", $newPassword, $token); $stmt->execute(); if ($stmt->affected_rows > 0) { echo "Password updated successfully!"; } else { echo "Invalid or expired token!"; } }