In this tutorial, we are going to learn Send email with attachment in PHP. We already seen mail sending functionality using SwiftMailer. attachment mail is implement in many forms like contact, inquiry and send custom invoice to customer. We are implement with helps of PHPMailer because it’s more secure compare to PHP Mail() function, support SMTP, attachment handling, easy to use and efficient.
We can design contact form as example and implement process of Secure email sending in PHP. In this PHP mail script with attachment example script covers code examples of contact form, SMTP setup, file uploads and file validation
Step-by-Step Guide to Send Emails with Attachments in PHP
✅ Step 1: Install PHPMailer
Install Composer and get PHPMailer lib from Github
composer require phpmailer/phpmailer
✅ Step 2: Create the PHP Contact Form
Below Contact form accepts the user input and file uploads. Use enctype="multipart/form-data"
for file handling.
<html> <head> <link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous"> </head> <body> <section class="jumbotron text-center"> <div class="container"> <h1 class="jumbotron-heading">CONTACT US</h1> </div> </section> <div class="container"> <div class="row"> <div class="col"> <?php echo isset($msg)? $msg : ""; ?> <div class="card"> <div class="card-header bg-primary text-white"><i class="fa fa-envelope"></i> Contact us. </div> <div class="card-body"> <form method="post" action="" enctype="multipart/form-data"> <div class="form-group"> <label for="name">Name</label> <input type="text" class="form-control" name="name" id="name" aria-describedby="emailHelp" placeholder="Enter name" required> </div> <div class="form-group"> <label for="email">Email address</label> <input type="email" class="form-control" name="email" id="email" aria-describedby="emailHelp" placeholder="Enter email" required> <small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small> </div> <div class="form-group"> <label for="message">Message</label> <textarea class="form-control" name="message" id="message" rows="6" required></textarea> </div> <div class="form-group"> <label for="attachment">Attachment</label> <input class="form-control" type="file" name="file" required><br><br> </div> <div class="mx-auto"> <input type="submit" name="submit" class="btn btn-primary text-right" value="SUBMIT"> </div> </form> </div> </div> </div> </div> </div> </body> </html>
✅ Step 3: Configure PHPMailer in PHP
Create file send_mail.php and include this on file.
<?php // Include the PHPMailer library use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; // Autoload PHPMailer require 'vendor/autoload.php'; // If using Composer, adjust the path if necessary $msg=$msgdiv=""; $from_email="recipient@domain.com"; $from_name = "Recipient Name"; if ($_SERVER["REQUEST_METHOD"] == "POST") { // Collect form data $name = $_POST['name']; $email = $_POST['email']; $message = $_POST['message']; $file = $_FILES['file']; // File details $file_name = $_FILES['file']['name']; $file_tmp = $_FILES['file']['tmp_name']; $file_type = $_FILES['file']['type']; $file_size = $_FILES['file']['size']; // Check if the file size is within the limit (e.g., 2MB) if ($file_size > 2097152) { // 2MB die('File size is too large. Maximum allowed size is 2MB.'); } // Create a new PHPMailer instance $mail = new PHPMailer(true); try { // Set mailer to use SMTP $mail->isSMTP(); $mail->Host = HOST; // Replace with your SMTP server $mail->SMTPAuth = true; $mail->Username = USERNAME; // SMTP username $mail->Password = PASSWORD; // SMTP password $mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS; $mail->Port = 587; // SMTP port (use 465 for SSL) // Set sender and recipient $mail->setFrom($email, $name); $mail->addAddress($from_email, $from_name); // Replace with your recipient // Set email format to HTML $mail->isHTML(true); $mail->Subject = 'New Form Submission with Attachment'; $mail->Body = "Name: $name<br>Email: $email <br> $message"; // Attach the uploaded file $mail->addAttachment($file_tmp, $file_name); // Send the email if ($mail->send()) { $msg='<div class="alert alert-success" role="alert">Email sent successfully! </div>'; $msgdiv="success"; } else { $msg='<div class="alert alert-danger" role="alert">Failed to send email.</div>'; $msgdiv="fail"; } } catch (Exception $e) { $msg='<div class="alert alert-danger" role="alert">Message could not be sent. Mailer Error: '.$mail->ErrorInfo.'</div>'; $msgdiv="fail"; } } ?>
✅ Step 4: PHPMailer Setup
- Use PHPMailer and Exception classes, for sending mail and Error handling.
- $mail->isSMTP() Send mail thought using SMTP Protocol.
- HOST, USERNAME and PASSWORD for SMTP Mail detail.
$mail->setFrom()
Set From mail$mail->addAddress()
. Set recipient’s email- $mail->isHTML(true) Which specify the Email send by HTML Format.
- $mail->addAttachment() Send Attachment by passing temporary file path and original path.
- $mail->send() Send mail to recipient and it return true or false.
✅ Step 5: Securely send emails with PHPMailer
- Restrict file types and sizes by adding validation rules.
- Store SMTP Credentials ON .env file which prevents for Stolen
- Enable SPF, DKIM, and DMARC for better email deliverability and spamming rate.
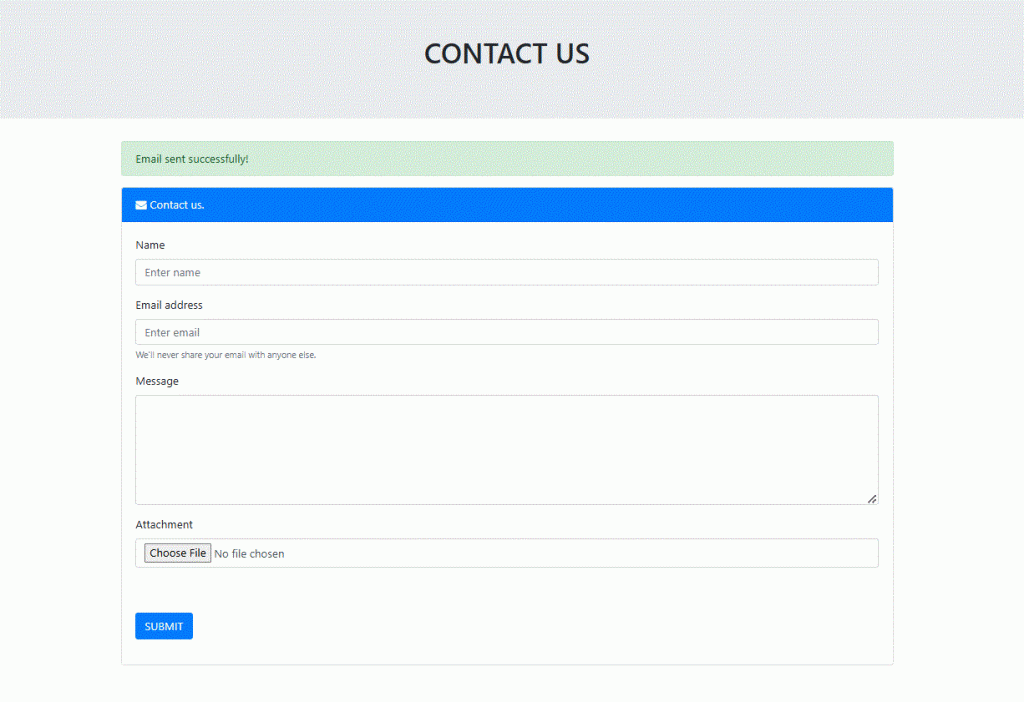
✅ Step 6: Troubleshooting Common Issues
- Email Not Sending?
Enable $mail->SMTPDebug = 2;
to debug issues.
Use correct SMTP Credentials.
- Emails Going to Spam?
Ensure Server setup proper SPF, DKIM, and DMARC
Ensure You have verified domain
Do Not use simple mail() function.
Conclusion
In our code we are using contact form as sending attachment email. As PHPMailer is secure and Professionally handle email templates. We are trying to explain everything in details like Validations, Debugging and Setup SMTP.